Python Versions
Don’t skip this, it’s important to understand this because a lot of confusion and errors stem from not understanding python versions and code compatibility.
Official Python has two different versions that were updated in parallel, Python 2.x and Python 3.x.
The reason for this split is because Python 3 code is not compatible with Python 2, that means code written for Python 2 will not work without changes in Python 3.
This issue causes a lot of the legacy code that was written in python 2 to be stuck in python 2.
Python 2 has officially been declared as no longer supported, and no more updates will be developed for it, therefore if you are learning Python now, you should be learning Python 3.
But be aware that some Linux systems and old scripts and guides still rely on Python 2.
The fastest way to recognize code meant for Python 2 is by looking at “print” commands.
If it looks like this: ‘print “Hello World!”‘ it’s python 2 code.
If it looks like this: ‘print(“Hello World!”)’ it’s probably python 3 code.
Installing Python / Checking Version
Linux
Most Linux distributions come with Python preinstalled.
Some distributions include Python 3, most distributions include Python 2.
Check if you have Python installed, and which version it is using:
$ python -V Python 2.7.12 $ python3 -V Python 3.5.2
Your python versions may vary, but in general a python -V command should return a 2.X version, and a python3 -V command should return a 3.X version.
If the python3 command did not work, you are missing python 3 and should install it with your distribution’s package manager.
For Ubuntu this is:
$ sudo apt install python3
When installation is complete double check the python3 -V command.
Windows
Windows does not come with Python preinstalled, you need to install it yourself.
It’s important to note where the installation is placing the installed files, and it’s VERY important to tell the installer to add Python to windows PATH, otherwise running Python on windows will not be as easy as just typing “python”.
Download latest version of Python 3.X from https://www.python.org/downloads/ and install it on your machine.
When you are done installing it, the following commands should work:
> where python C:\...\python.exe > python -V Python 3.8.2
Visual Studio Code
Visual Studio Code is an Integrated Development Environment used to write code in a large variety of languages, including Python.
Note that this is not Visual Studio, which is a completely different product.
Visual Studio Code is lightweight, feature full and available on most platforms, and its free.
Download and install it from https://code.visualstudio.com/.
When done installing, create a folder for your projects.
Recommendation for Windows: C:\Users\<user>\Projects or Desktop\Projects
Recommendation for Linux: ~/projects
Now create a folder for your first project, “helloworld“.
Now start Visual Studio Code from that folder. (Linux and Windows commands below)
user@localhost:~/projects$ code helloworld C:\Users\User> code helloworld
This should open Visual Studio Code, with an empty file list on the left side.
Hello World
To make sure everything is working fine let’s make our first python program, run it and see that it works as expected.
Visual Studio Code has keyboard shortcuts, and this tutorial will be using them as much as possible.
Create a new file in your working folder by pressing Ctrl+N.
Write the following in the newly created file:
print("Hello World!")
Save the file using Ctrl+S, name it “hello.py” (Make sure its saved in your projects folder)
Once this is done, VSCode will highlight syntax for this file, and it will probably ask you if you want to install python extension, install it.
Once it’s done it will also ask about “pylint” linter not being installed, either install that ,or select a different linter (flake8 is a good one) and install it.
A linter is an additional program/script that goes over your code and highlights style issues, or suggestions based on the accepted coding style PEP8 ( https://www.python.org/dev/peps/pep-0008/ )
It’s a good practice to listen to it’s suggestions and correct errors it finds.
Once this is done, you should have a python file named “hello.py” open in the main window, and it should show up on the left side.
Running the code is done with Ctrl+F5 keyboard shortcut, if everything went right you should see the output on the bottom of the screen.
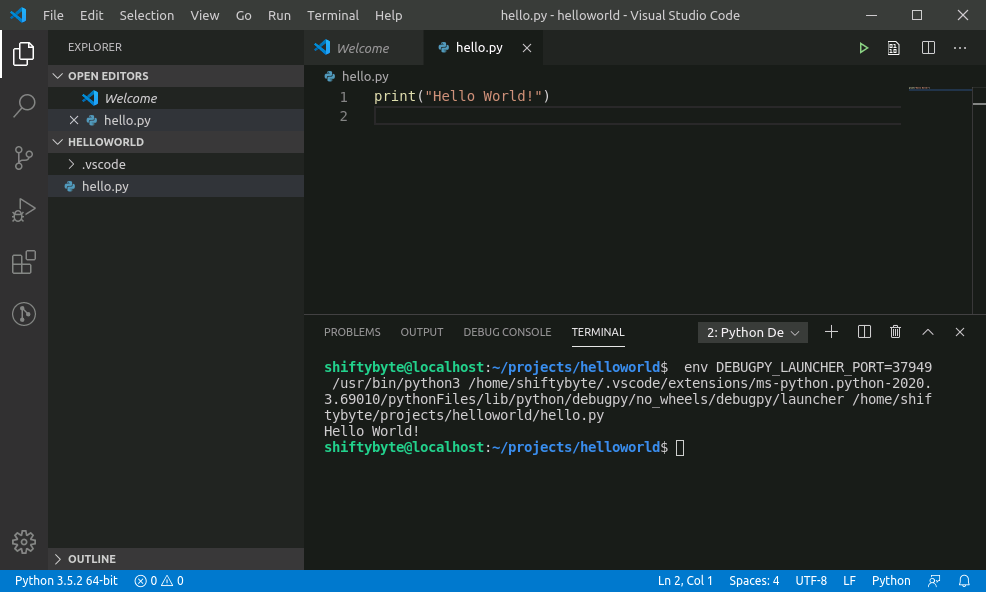